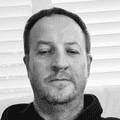
Full Stack Engineer
Introducing the AWS Cloud Development Kit
AWS CDK is an open-source framework that lets you create and provision AWS resources using code. At the time of writing, AWS CDK supports TypeScript, JavaScript, Python, Java, C#/.Net, and Go.
Key concepts
The AWS CDK is designed around a handful of important concepts. I will introduce a few of these here briefly.
An AWS CDK app is an application written in one of the languages mentioned above. An app defines one or more stacks. Stacks (Essentially AWS CloudFormation stacks). Stacks are comprised of Constructs which are cloud components (think Lambda Functions, S3 buckets, SQS queues etc).
The AWS CDK includes a CLI toolkit (Command Line Interface) for working with your AWS CDK apps. The CLI allows you to perform tasks such as:
- Convert AWS CDK stacks to AWS CloudFormation templates
- Deploy your stacks to an AWS account and Region
To install the toolkit you can simply use the following command:
npm install -g aws-cdk
And then you can use the toolkit to create a new typescript project:
- Template - can be
app
,lib
andsample-app
- Language - can be
TypeScript
,JavaScript
,Python
,Java
,C#/.Net
, andGo
.
cdk init template --language language
For a full list of commands that are contained in the CLI you can just use:
cdk help
Constructs
The AWS CDK includes a library of AWS constructs. Each AWS service is represented by a construct. Constructs are defined by levels. There are 3 levels:
L1 (Level 1)
These constructs correspond directly to resource types defined by AWS CloudFormation. These constructs are prefixed with Cfn
before the construct name.
An example L1 construct:
// The code below shows an example of how to instantiate this type.
// The values are placeholders you should change.
import { aws_apigateway as apigateway } from 'aws-cdk-lib';
const cfnApiKey = new apigateway.CfnApiKey(
this,
'MyCfnApiKey',
/* all optional props */ {
customerId: 'customerId',
description: 'description',
enabled: false,
generateDistinctId: false,
name: 'name',
stageKeys: [
{
restApiId: 'restApiId',
stageName: 'stageName',
},
],
tags: [
{
key: 'key',
value: 'value',
},
],
value: 'value',
},
);
L2 (Level 2)
These constructs are developed by the AWS CDK team for specific use cases and make it easier to create simple infrastructure. L2 constructs (in most cases) encapsulate L1 resources.
An example L2 construct:
this.api = new apigateway.RestApi(this, 'ApiGatewayRestApi', {
defaultCorsPreflightOptions: {
allowOrigins: apigateway.Cors.ALL_ORIGINS,
allowMethods: apigateway.Cors.ALL_METHODS,
},
});
this.api.root.addMethod(
'POST',
new apigateway.LambdaIntegration(this.lambdaFunction, {
proxy: true,
}),
);
L3 (Level 3)
Level 3 constructs are patterns. These constructs allow easy configuration of resources, grant permissions etc.
An example L3 construct:
const restApi = new apigateway.LambdaRestApi(this, 'LambdaRestApiL3', {
handler: this.lambdaFunction,
defaultCorsPreflightOptions: {
allowOrigins: apigateway.Cors.ALL_ORIGINS,
allowMethods: apigateway.Cors.ALL_METHODS,
},
});
This is only a small introduction to constructs. You can find more examples in the api docs or construct hub
Getting started
Installing the CDK via npm:
npm install -g aws-cdk
Verify the installation:
cdk help
Bootstrapping the environment:
cdk bootstrap aws://aws-acccount-number/aws-region
Bootstrapping is the process of provisioning resources for the AWS CDK before you can deploy AWS CDK apps into an AWS environment.
Create a new app:
cdk init app --language typescript
Deploy the stack:
cdk deploy
I hope you enjoyed the article which is a small introduction to the AWS CDK. There's plenty of information that I didn't cover here. You can read more about the AWS CDK here